Text column
Overview
Text columns display simple text from your database:
use Filament\Tables\Columns\TextColumn;
TextColumn::make('title')
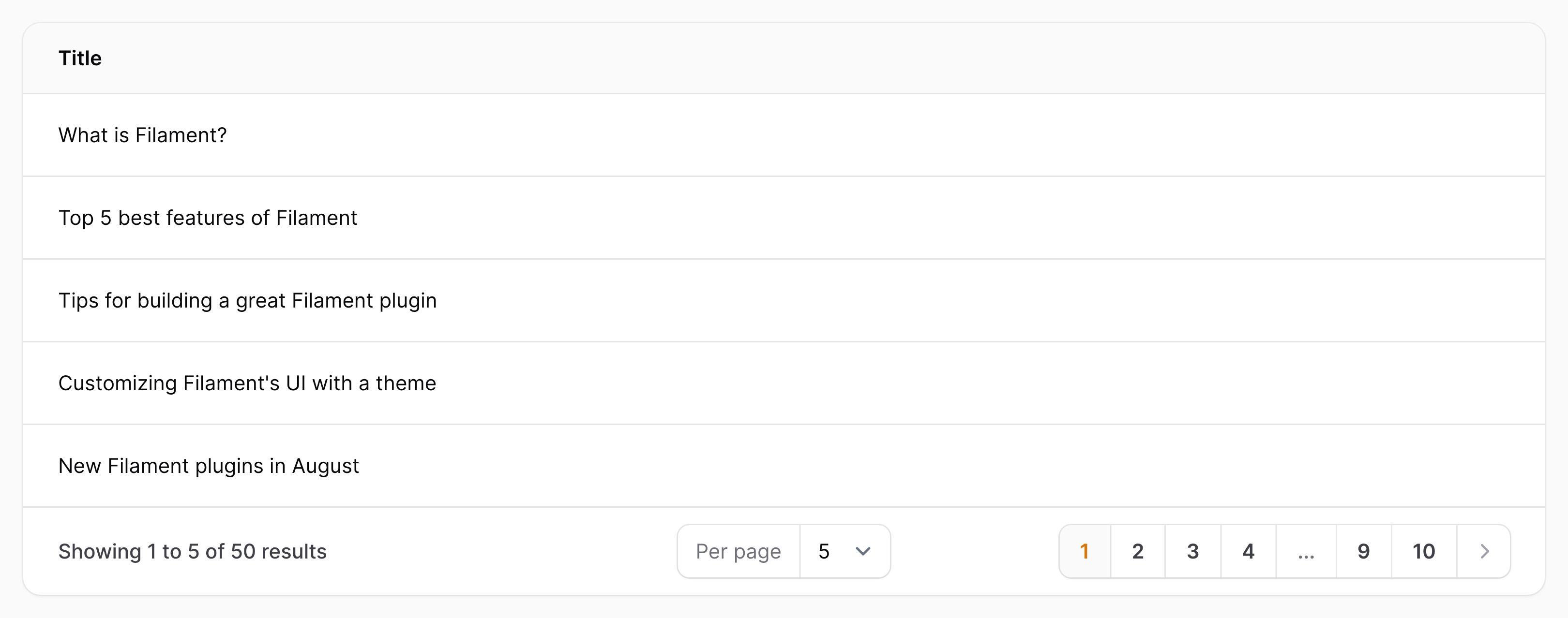
Displaying as a "badge"
By default, text is quite plain and has no background color. You can make it appear as a "badge" instead using the badge()
method. A great use case for this is with statuses, where may want to display a badge with a color that matches the status:
use Filament\Tables\Columns\TextColumn;
TextColumn::make('status')
->badge()
->color(fn (string $state): string => match ($state) {
'draft' => 'gray',
'reviewing' => 'warning',
'published' => 'success',
'rejected' => 'danger',
})
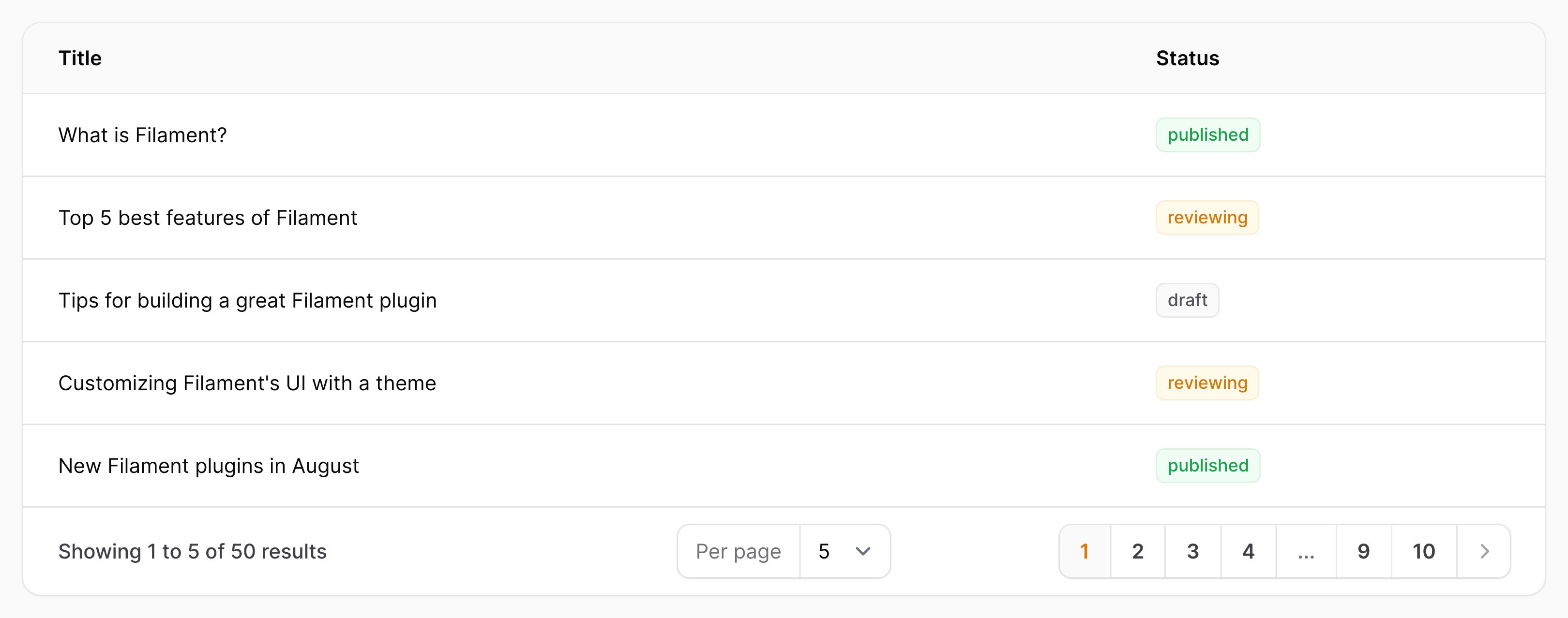
You may add other things to the badge, like an icon.
Displaying a description
Descriptions may be used to easily render additional text above or below the column contents.
You can display a description below the contents of a text column using the description()
method:
use Filament\Tables\Columns\TextColumn;
TextColumn::make('title')
->description(fn (Post $record): string => $record->description)
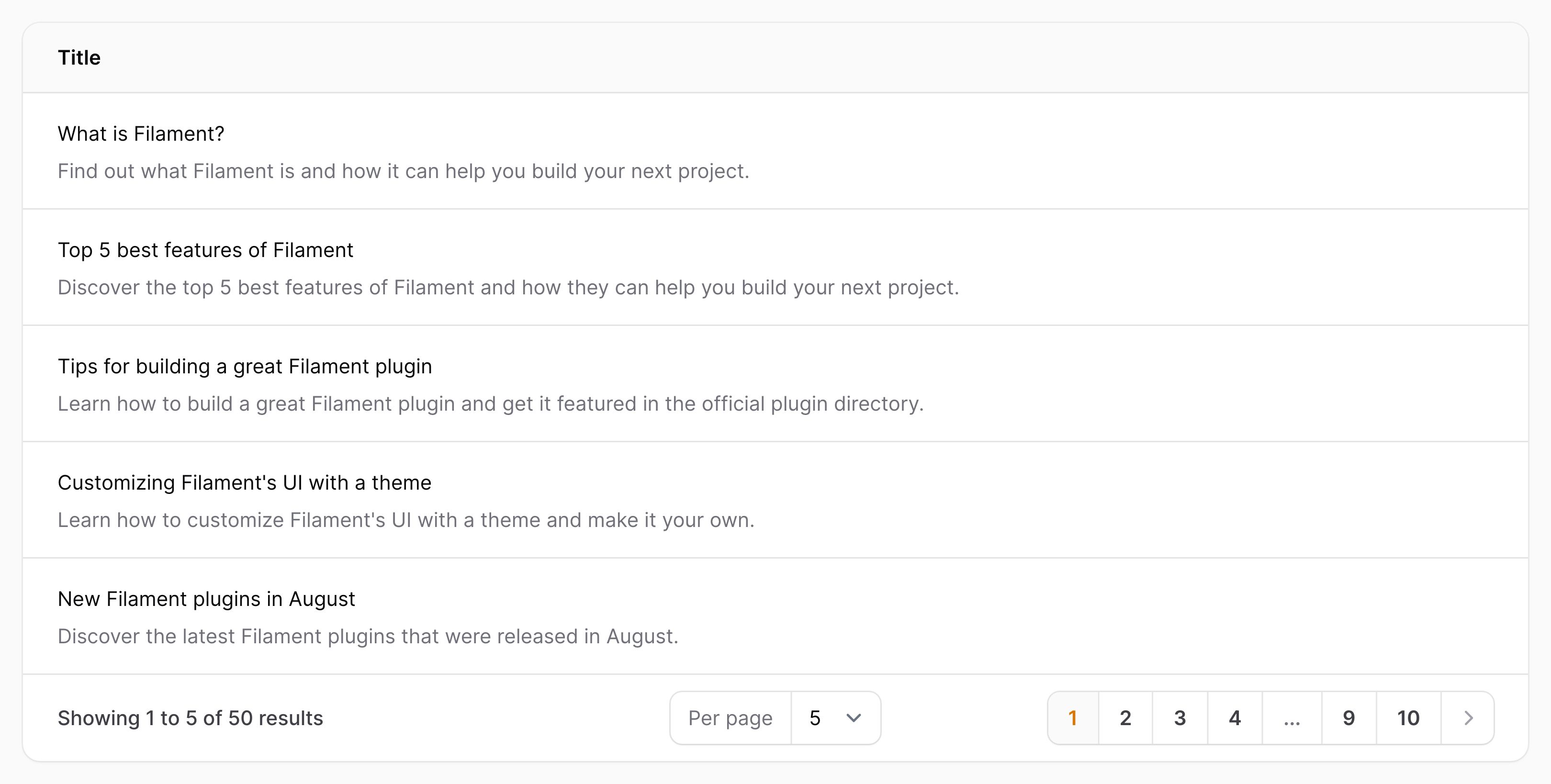
By default, the description is displayed below the main text, but you can move it above using the second parameter:
use Filament\Tables\Columns\TextColumn;
TextColumn::make('title')
->description(fn (Post $record): string => $record->description, position: 'above')
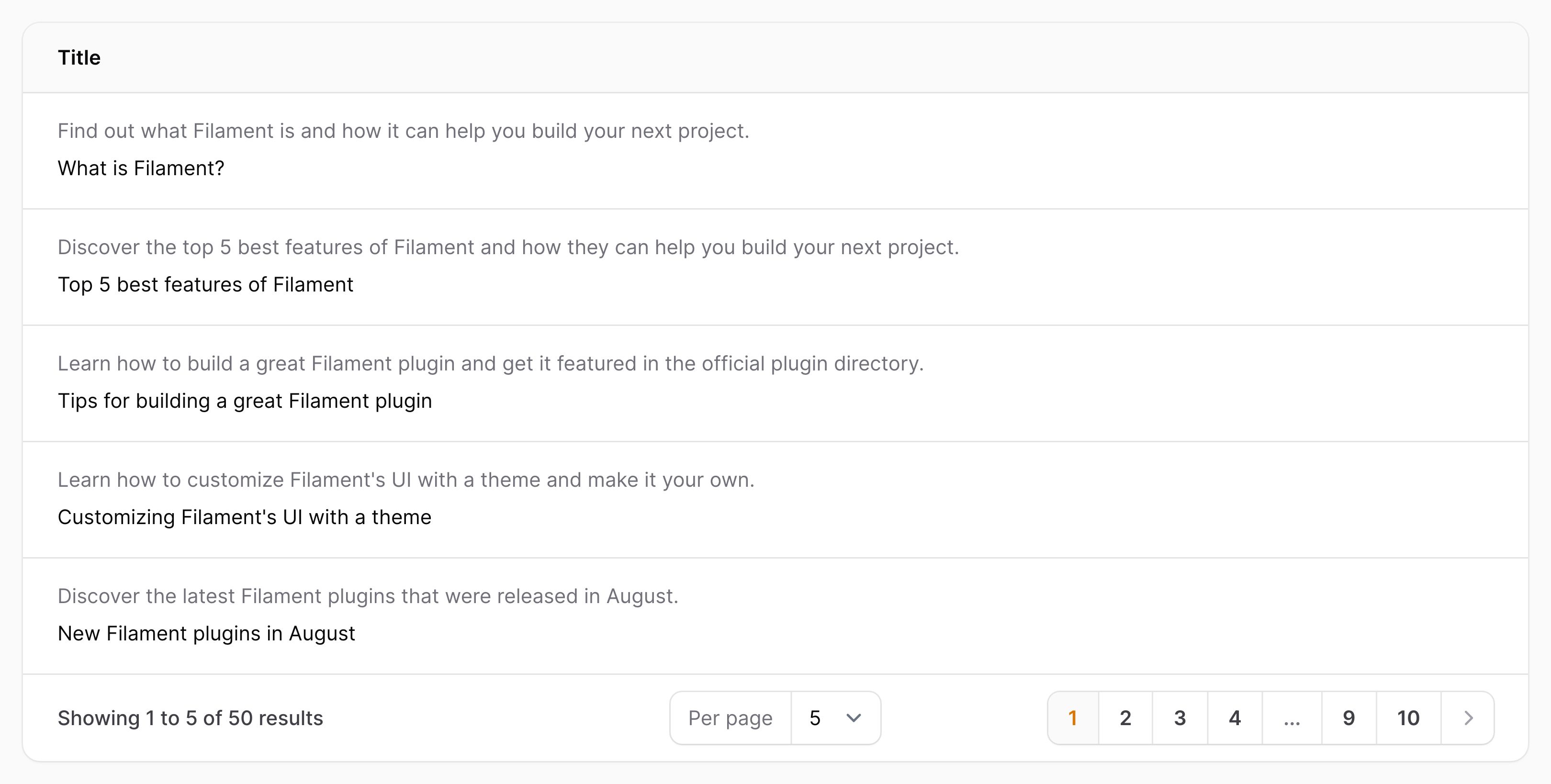
Date formatting
You may use the date()
and dateTime()
methods to format the column's state using PHP date formatting tokens:
use Filament\Tables\Columns\TextColumn;
TextColumn::make('created_at')
->dateTime()
You may use the since()
method to format the column's state using Carbon's diffForHumans()
:
use Filament\Tables\Columns\TextColumn;
TextColumn::make('created_at')
->since()
Number formatting
The numeric()
method allows you to format a column as a number, using PHP's number_format()
:
use Filament\Tables\Columns\TextColumn;
TextColumn::make('stock')
->numeric(
decimalPlaces: 0,
decimalSeparator: '.',
thousandsSeparator: ',',
)
Currency formatting
The money()
method allows you to easily format monetary values, in any currency:
use Filament\Tables\Columns\TextColumn;
TextColumn::make('price')
->money('EUR')
Limiting text length
You may limit()
the length of the cell's value:
use Filament\Tables\Columns\TextColumn;
TextColumn::make('description')
->limit(50)
You may also reuse the value that is being passed to limit()
:
use Filament\Tables\Columns\TextColumn;
TextColumn::make('description')
->limit(50)
->tooltip(function (TextColumn $column): ?string {
$state = $column->getState();
if (strlen($state) <= $column->getCharacterLimit()) {
return null;
}
// Only render the tooltip if the column content exceeds the length limit.
return $state;
})
Limiting word count
You may limit the number of words()
displayed in the cell:
use Filament\Tables\Columns\TextColumn;
TextColumn::make('description')
->words(10)
Wrapping content
If you'd like your column's content to wrap if it's too long, you may use the wrap()
method:
use Filament\Tables\Columns\TextColumn;
TextColumn::make('description')
->wrap()
Listing multiple values
By default, if there are multiple values inside your text column, they will be comma-separated. You may use the listWithLineBreaks()
method to display them on new lines instead:
use Filament\Tables\Columns\TextColumn;
TextColumn::make('authors.name')
->listWithLineBreaks()
Adding bullet points to the list
You may add a bullet point to each list item using the bulleted()
method:
use Filament\Tables\Columns\TextColumn;
TextColumn::make('authors.name')
->listWithLineBreaks()
->bulleted()
Limiting the number of values in the list
You can limit the number of values in the list using the limitList()
method:
use Filament\Tables\Columns\TextColumn;
TextColumn::make('authors.name')
->listWithLineBreaks()
->limitList(3)
Using a list separator
If you want to "explode" a text string from your model into multiple list items, you can do so with the separator()
method. This is useful for displaying comma-separated tags as badges, for example:
use Filament\Tables\Columns\TextColumn;
TextColumn::make('tags')
->badge()
->separator(',')
Rendering HTML
If your column value is HTML, you may render it using html()
:
use Filament\Tables\Columns\TextColumn;
TextColumn::make('description')
->html()
Rendering Markdown as HTML
If your column contains Markdown, you may render it using markdown()
:
use Filament\Tables\Columns\TextColumn;
TextColumn::make('description')
->markdown()
Custom formatting
You may instead pass a custom formatting callback to formatStateUsing()
, which accepts the $state
of the cell, and optionally its $record
:
use Filament\Tables\Columns\TextColumn;
TextColumn::make('status')
->formatStateUsing(fn (string $state): string => __("statuses.{$state}"))
Customizing the color
You may set a color for the text, either danger
, gray
, info
, primary
, success
or warning
:
use Filament\Tables\Columns\TextColumn;
TextColumn::make('status')
->color('primary')

Adding an icon
Text columns may also have an icon:
use Filament\Tables\Columns\TextColumn;
TextColumn::make('email')
->icon('heroicon-m-envelope')

You may set the position of an icon using iconPosition()
:
use Filament\Support\Enums\IconPosition;
use Filament\Tables\Columns\TextColumn;
TextColumn::make('email')
->icon('heroicon-m-envelope')
->iconPosition(IconPosition::After) // `IconPosition::Before` or `IconPosition::After`
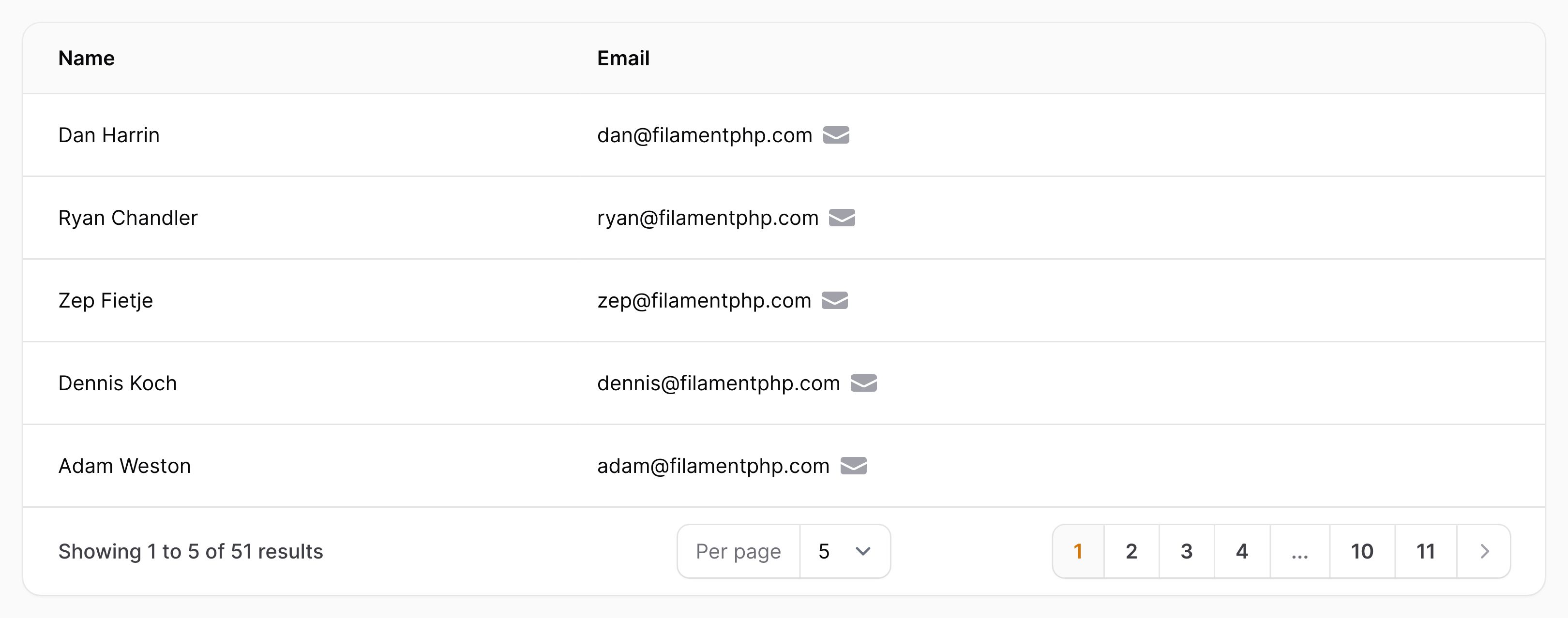
Customizing the text size
You may make the text larger using size(TextColumnSize::Large)
:
use Filament\Tables\Columns\TextColumn;
TextColumn::make('title')
->size(TextColumn\TextColumnSize::Large)

Customizing the font weight
Text columns have regular font weight by default, but you may change this to any of the following options: FontWeight::Thin
, FontWeight::ExtraLight
, FontWeight::Light
, FontWeight::Medium
, FontWeight::SemiBold
, FontWeight::Bold
, FontWeight::ExtraBold
or FontWeight::Black
.
For instance, you may make the font bold using weight(FontWeight::Bold)
:
use Filament\Support\Enums\FontWeight;
use Filament\Tables\Columns\TextColumn;
TextColumn::make('title')
->weight(FontWeight::Bold)

Customizing the font family
You can change the text font family to any of the following options: FontFamily::Sans
, FontFamily::Serif
or FontFamily::Mono
.
For instance, you may make the font mono using fontFamily(FontFamily::Mono)
:
use Filament\Support\Enums\FontFamily;
use Filament\Tables\Columns\TextColumn;
TextColumn::make('email')
->fontFamily(FontFamily::Mono)

Allowing the text to be copied to the clipboard
You may make the text copyable, such that clicking on the cell copies the text to the clipboard, and optionally specify a custom confirmation message and duration in milliseconds. This feature only works when SSL is enabled for the app.
use Filament\Tables\Columns\TextColumn;
TextColumn::make('email')
->copyable()
->copyMessage('Email address copied')
->copyMessageDuration(1500)

Customizing the text that is copied to the clipboard
You can customize the text that gets copied to the clipboard using the copyableState()
method:
use Filament\Tables\Columns\TextColumn;
TextColumn::make('url')
->copyable()
->copyableState(fn (string $state): string => "URL: {$state}")
In this function, you can access the whole table row with $record
:
use App\Models\Post;
use Filament\Tables\Columns\TextColumn;
TextColumn::make('url')
->copyable()
->copyableState(fn (Post $record): string => "URL: {$record->url}")
Displaying the row index
You may want a column to contain the number of the current row in the table:
use Filament\Tables\Columns\TextColumn;
use Filament\Tables\Contracts\HasTable;
TextColumn::make('index')->state(
static function (HasTable $livewire, stdClass $rowLoop): string {
return (string) (
$rowLoop->iteration +
($livewire->getTableRecordsPerPage() * (
$livewire->getTablePage() - 1
))
);
}
),
As $rowLoop
is Laravel Blade's $loop
object, you can reference all other $loop
properties.
As a shortcut, you may use the rowIndex()
method:
use Filament\Tables\Columns\TextColumn;
TextColumn::make('index')
->rowIndex()
To start counting from 0 instead of 1, use isFromZero: true
:
use Filament\Tables\Columns\TextColumn;
TextColumn::make('index')
->rowIndex(isFromZero: true)