Text column
Overview
Text columns display simple text from your database:
use Filament\Tables\Columns\TextColumn;
TextColumn::make('title')
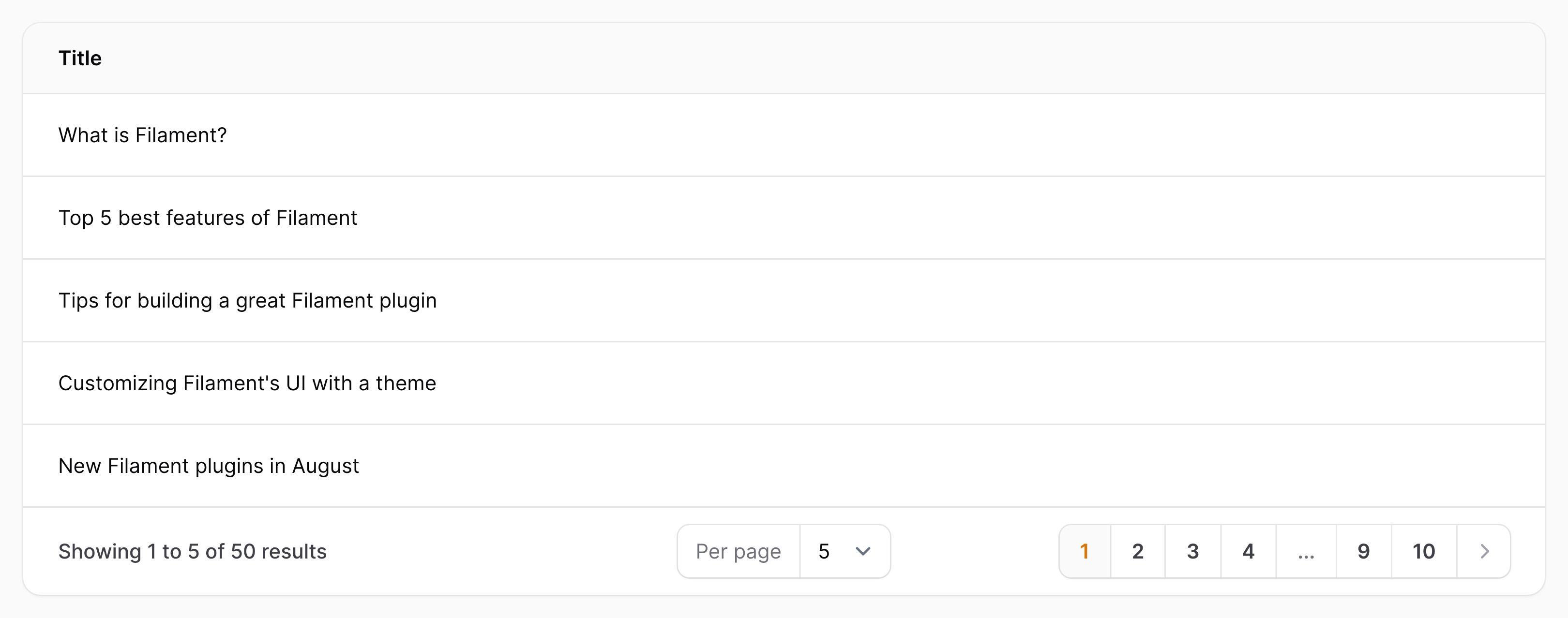
Displaying as a "badge"
By default, the text is quite plain and has no background color. You can make it appear as a "badge" instead using the badge()
method. A great use case for this is with statuses, where may want to display a badge with a color that matches the status:
use Filament\Tables\Columns\TextColumn;
TextColumn::make('status')
->badge()
->color(fn (string $state): string => match ($state) {
'draft' => 'gray',
'reviewing' => 'warning',
'published' => 'success',
'rejected' => 'danger',
})
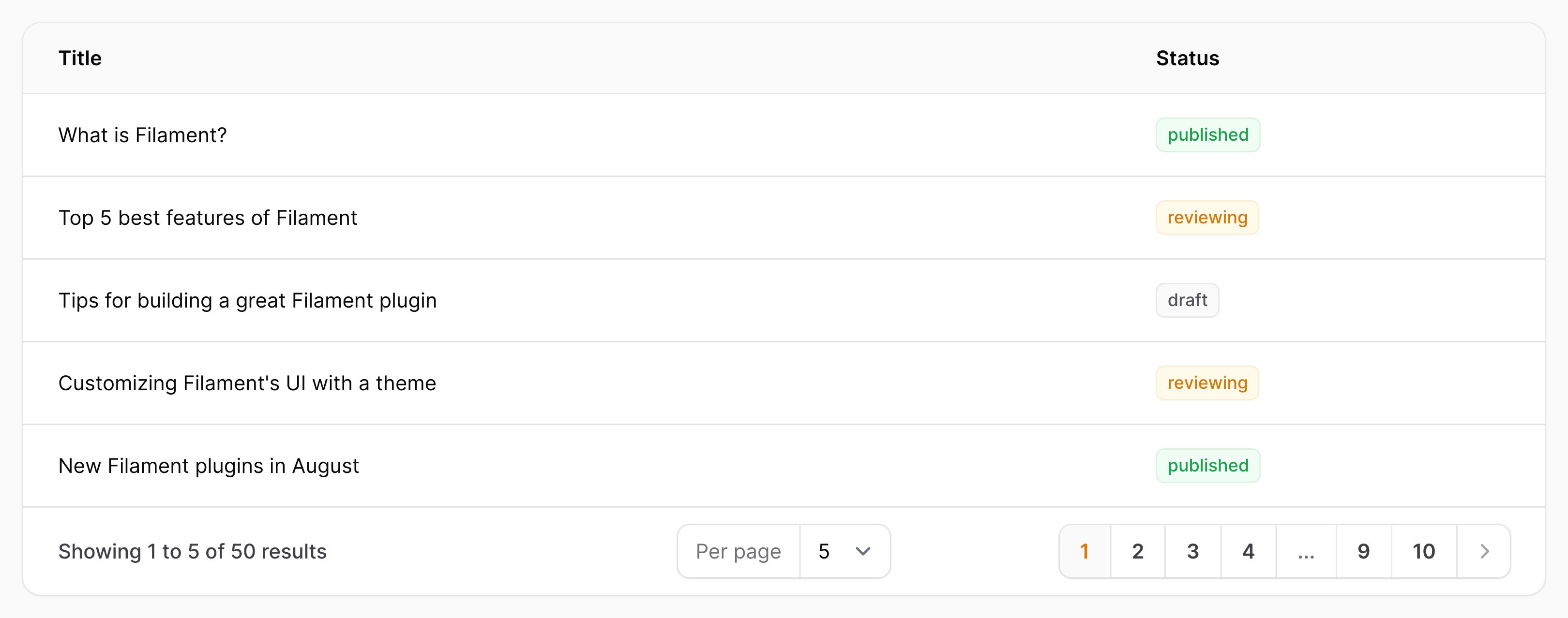
You may add other things to the badge, like an icon.
Displaying a description
Descriptions may be used to easily render additional text above or below the column contents.
You can display a description below the contents of a text column using the description()
method:
use Filament\Tables\Columns\TextColumn;
TextColumn::make('title')
->description(fn (Post $record): string => $record->description)
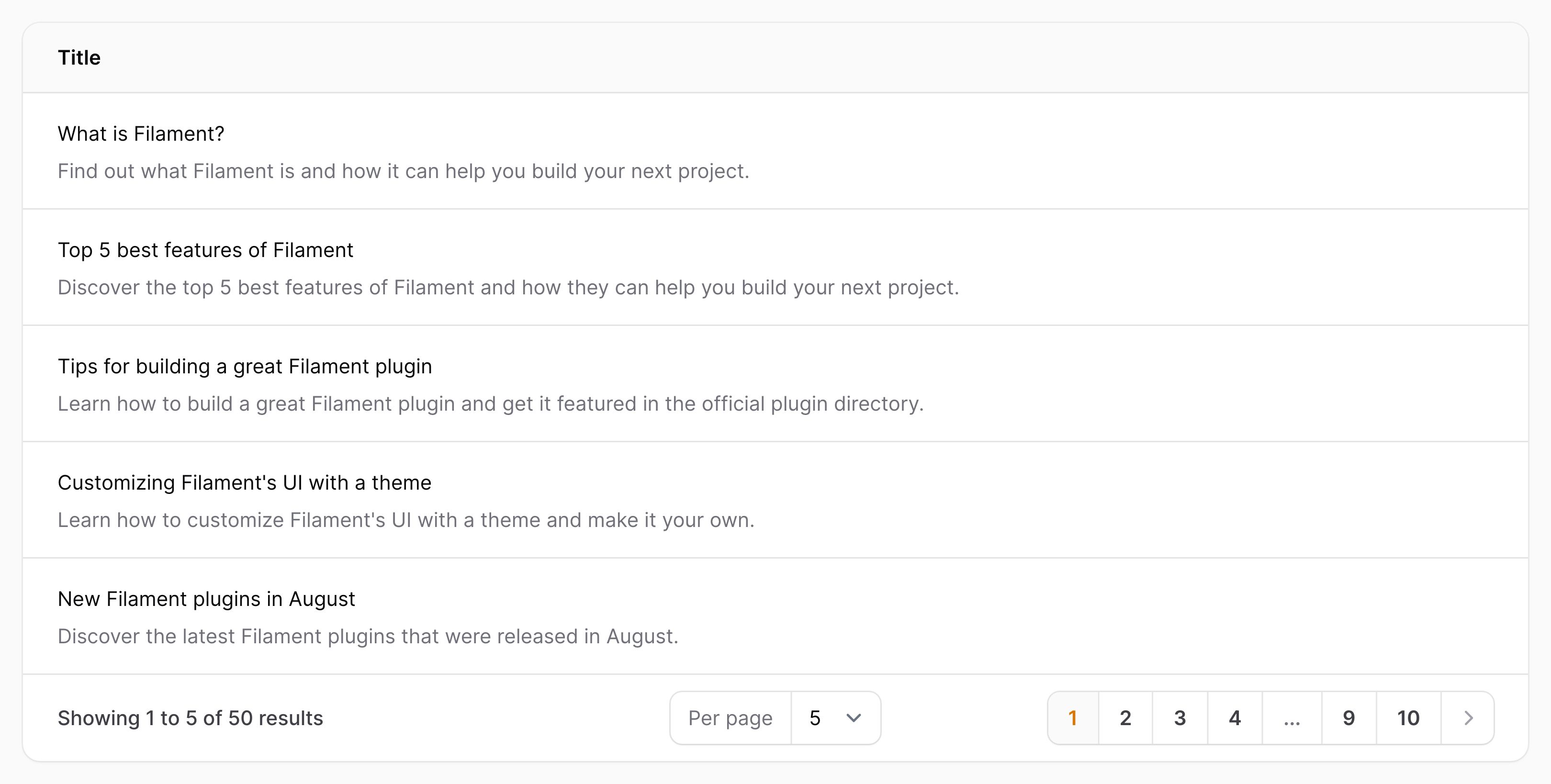
By default, the description is displayed below the main text, but you can move it above using the second parameter:
use Filament\Tables\Columns\TextColumn;
TextColumn::make('title')
->description(fn (Post $record): string => $record->description, position: 'above')
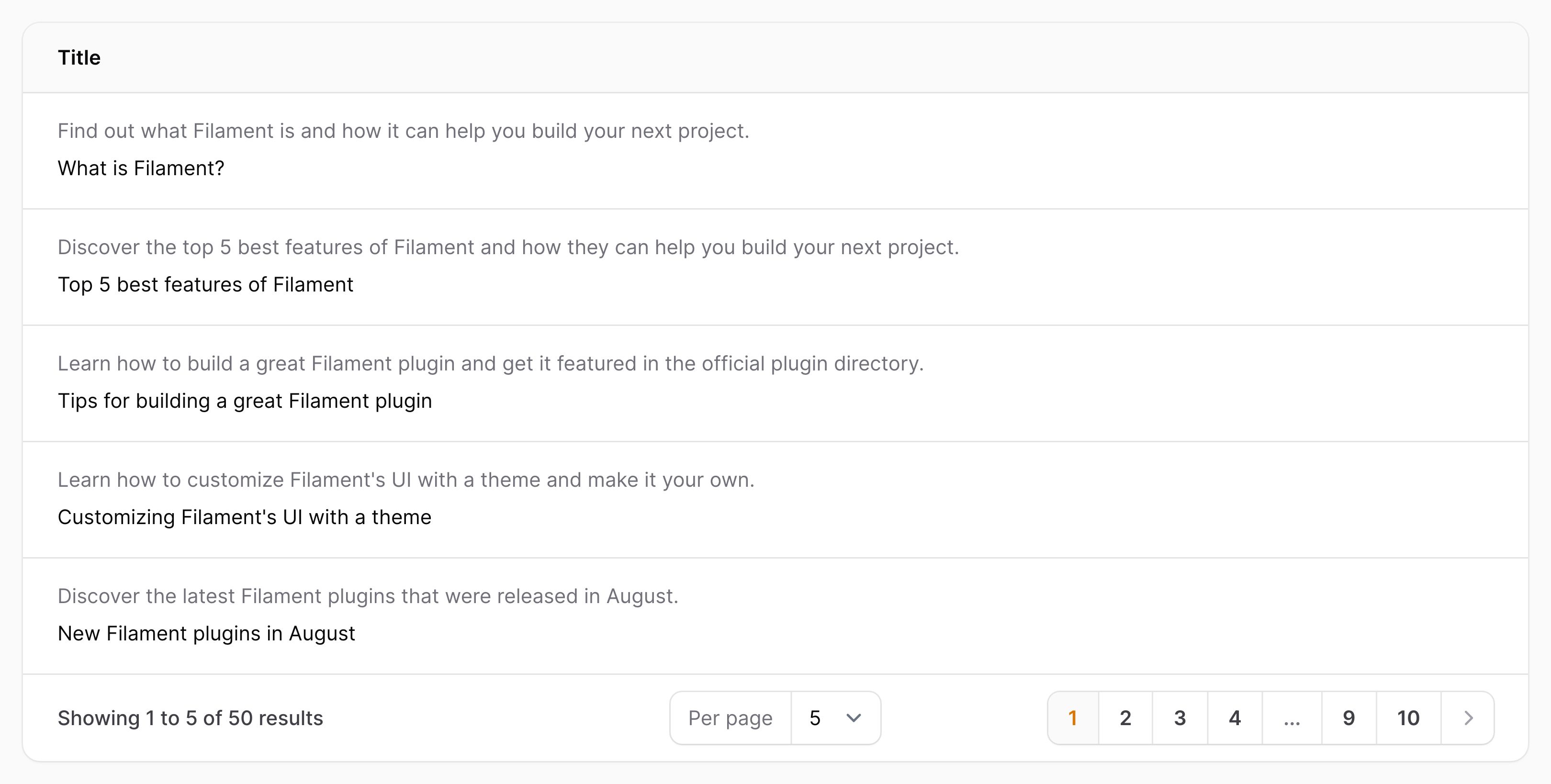
Date formatting
You may use the date()
and dateTime()
methods to format the column's state using PHP date formatting tokens:
use Filament\Tables\Columns\TextColumn;
TextColumn::make('created_at')
->dateTime()
You may use the since()
method to format the column's state using Carbon's diffForHumans()
:
use Filament\Tables\Columns\TextColumn;
TextColumn::make('created_at')
->since()
Number formatting
The numeric()
method allows you to format an entry as a number:
use Filament\Tables\Columns\TextColumn;
TextColumn::make('stock')
->numeric()
If you would like to customize the number of decimal places used to format the number with, you can use the decimalPlaces
argument:
use Filament\Tables\Columns\TextColumn;
TextColumn::make('stock')
->numeric(decimalPlaces: 0)