模态框
概述
Action 可能会在运行前要求额外确认或者用户输入。你可以在 Action 执行之前打开模态框实现该功能。
确认模态框
使用 requiresConfirmation()
方法,你可以在 Action 运行之前请求确认。这对于破坏性操作,比如删除记录,特别有用。
Action::make('delete')
->action(fn () => $this->record->delete())
->requiresConfirmation()

如果使用
url()
代替action()
,那确认模态框不可用。相反,你应该在action()
方法内,重定向 URL。
模态框表单
你也可以在模态框中渲染表单,在 Action 运行之前从用户那边收集额外的信息。
你可以使用表单构造器中的组件来创建自定义模态框表单。表单中的数据可以通过 action()
闭包中的 $data
数组获取:
use App\Models\User;
use Filament\Forms\Components\Select;
Action::make('updateAuthor')
->form([
Select::make('authorId')
->label('Author')
->options(User::query()->pluck('name', 'id'))
->required(),
])
->action(function (array $data): void {
$this->record->author()->associate($data['authorId']);
$this->record->save();
})

使用现有数据填充表单
通过 fillForm()
方法,你可以使用现有数据填充表单:
use App\Models\User;
use Filament\Forms\Components\Select;
use Filament\Forms\Form;
Action::make('updateAuthor')
->fillForm([
'authorId' => $this->record->author->id,
])
->form([
Select::make('authorId')
->label('Author')
->options(User::query()->pluck('name', 'id'))
->required(),
])
->action(function (array $data): void {
$this->record->author()->associate($data['authorId']);
$this->record->save();
})
使用 Wizard 作为模态框表单
你可以在模态框中创建多步骤表单向导卡(wizard)。定义 step()
字符串,并传入 Step
对象:
use Filament\Forms\Components\MarkdownEditor;
use Filament\Forms\Components\TextInput;
use Filament\Forms\Components\Toggle;
use Filament\Forms\Components\Wizard\Step;
Action::make('create')
->steps([
Step::make('Name')
->description('Give the category a unique name')
->schema([
TextInput::make('name')
->required()
->live()
->afterStateUpdated(fn ($state, callable $set) => $set('slug', Str::slug($state))),
TextInput::make('slug')
->disabled()
->required()
->unique(Category::class, 'slug'),
])
->columns(2),
Step::make('Description')
->description('Add some extra details')
->schema([
MarkdownEditor::make('description'),
]),
Step::make('Visibility')
->description('Control who can view it')
->schema([
Toggle::make('is_visible')
->label('Visible to customers.')
->default(true),
]),
])
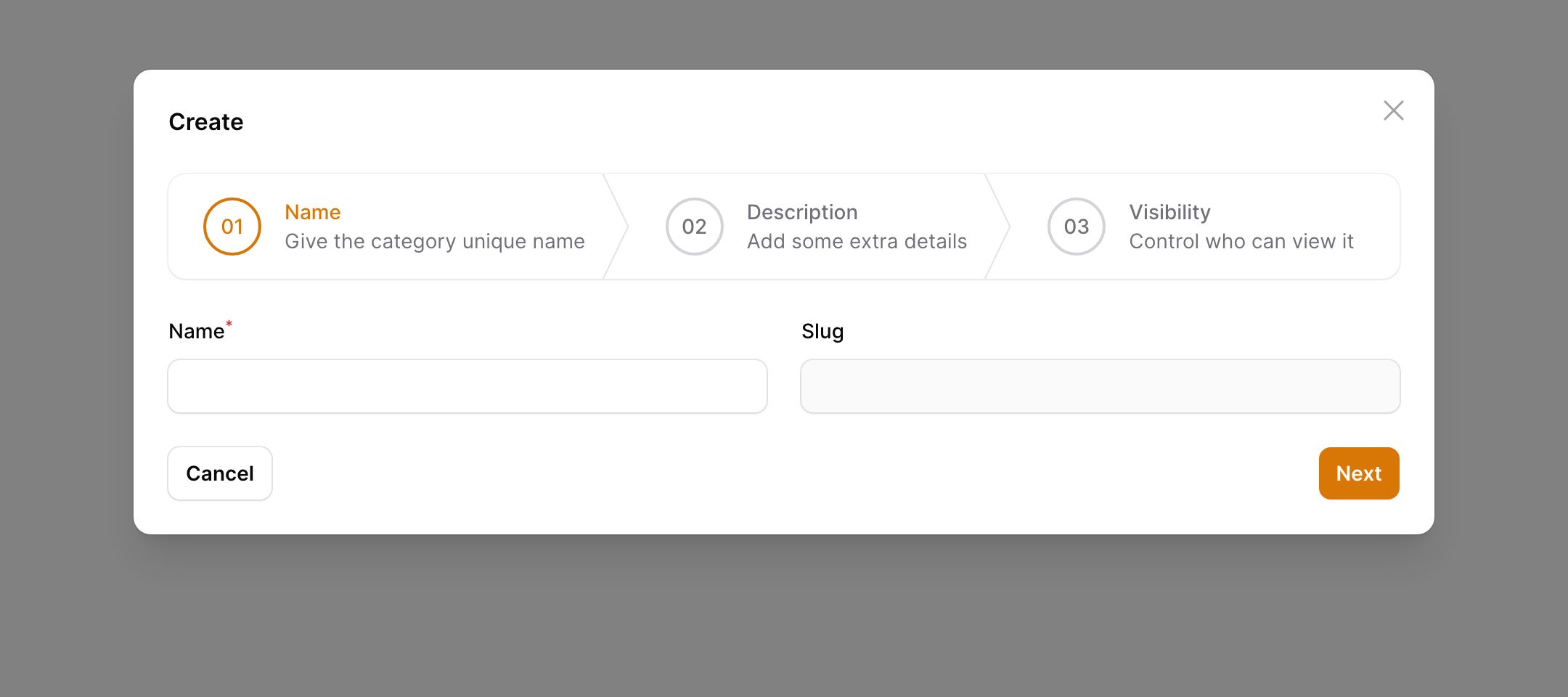
禁用所有表单字段
你可以使用 disabledForm()
方法,禁用模态框中的所有表单字段,确保用户不能对其进行编辑:
use App\Models\User;
use Filament\Forms\Components\Textarea;
use Filament\Forms\Components\TextInput;
Action::make('approvePost')
->form([
TextInput::make('title'),
Textarea::make('content'),
])
->fillForm([
'title' => $this->record->title,
'content' => $this->record->content,
])
->disabledForm()
->action(function (): void {
$this->record->approve();
})
自定义模态框标题、描述及按钮标签
你可以自定义模态框的标题、描述及提交按钮标签:
Action::make('delete')
->action(fn () => $this->record->delete())
->requiresConfirmation()
->modalHeading('Delete post')
->modalDescription('Are you sure you\'d like to delete this post? This cannot be undone.')
->modalSubmitActionLabel('Yes, delete it')
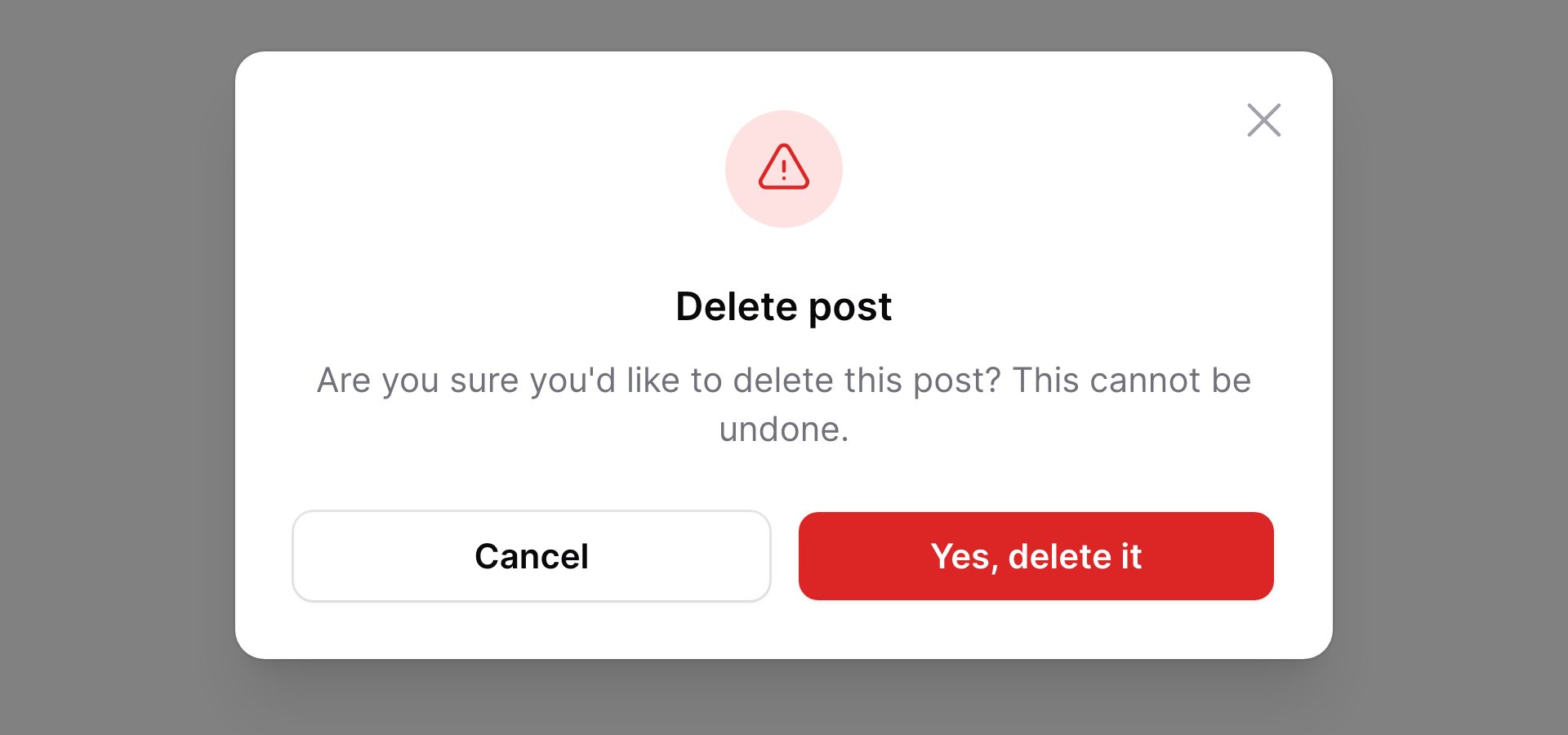
在模态框中添加图标
使用 modalIcon()
方法,你可以在模态框中添加图标:
Action::make('delete')
->action(fn () => $this->record->delete())
->requiresConfirmation()
->modalIcon('heroicon-o-trash')

默认情况下,图标继承了 Action 按钮的颜色。你可以使用 modalIconColor()
方法自定义图标颜色:
Action::make('delete')
->action(fn () => $this->record->delete())
->requiresConfirmation()
->color('danger')
->modalIcon('heroicon-o-trash')
->modalIconColor('warning')
自定义模态框内容的对齐方式
默认情况下,模态框内容对齐到起始位置,或者如果模态框宽度是 xs
或 sm
,则对齐到中间。如果你想修改模态框内容的对齐方式,可以在 modalAlignment()
中传入 Alignment::Start
或者 Alignment::Center
:
use Filament\Support\Enums\Alignment;
Action::make('updateAuthor')
->form([
// ...
])
->action(function (array $data): void {
// ...
})
->modalAlignment(Alignment::Center)
自定义模态框内容
你可以将 Blade 视图传入 modalContent()
函数,在模态框中渲染自定义内容。
Action::make('advance')
->action(fn () => $this->record->advance())
->modalContent(view('filament.pages.actions.advance'))
传递数据到自定义模态框内容
你可以通过返回函数,将数据传递到视图中。比如,如果设置了 Action 的 $record
,你可以将其传入到视图中:
use Illuminate\Contracts\View\View;
Action::make('advance')
->action(fn (Contract $record) => $record->advance())
->modalContent(fn (Contract $record): View => view(
'filament.pages.actions.advance',
['record' => $record],
))
在表单下添加自定义模态框内容
默认情况下,如果有自定义内容的话,它们会展示在模态框表单之上,不过你可以使用 modalContentFooter()
将内容添加到表单下面:
Action::make('advance')
->action(fn () => $this->record->advance())
->modalContentFooter(view('filament.pages.actions.advance'))
添加 Action 到自定义模态框内容
你可以添加 Action 按钮到自定义模态框内容,如果你想添加按钮执行一个 Action(非主 Action),这就有用。你可以使用 registerModalActions()
方法注册 Action,并将其传入到视图中:
use Illuminate\Contracts\View\View;
Action::make('advance')
->registerModalActions([
Action::make('report')
->requiresConfirmation()
->action(fn () => $this->record->report()),
])
->action(fn () => $this->record->advance())
->modalContent(fn (Action $action): View => view(
'filament.pages.actions.advance',
['action' => $action],
))
现在,在视图文件中,你可以调用 getModalAction()
来渲染 Action 按钮:
<div>
{{ $action->getModalAction('report') }}
</div>
使用 SlideOver 替代模态框
使用 slideOver()
方法,你可以打开 slide-over
对话框,而非模态框:
Action::make('updateAuthor')
->form([
// ...
])
->action(function (array $data): void {
// ...
})
->slideOver()

不再是在屏幕的中间打开,模态框内容现在会从右边滑入(slide in)并消费浏览器的全部高度。
粘性模态框 Header
当模态框内容超过模态框大小时,模态框的 Header 会滚动出屏幕。不过,SlideOver 使用了粘性模态框,使之总是可见。你可以使用 stickyModalHeader()
控制该行为:
Action::make('updateAuthor')
->form([
// ...
])
->action(function (array $data): void {
// ...
})
->stickyModalHeader()